Pre-requisites
- Before we get started, you’ll need to activate the preview feature “ParseJSON function and untyped objects” in your PowerApps settings. To do this, go to the Settings menu, browse to Upcoming features, and turn on the setting called ParseJSON function and untyped objects. If this feature is not turned on, the ParseJSON function will not be found in the formula bar.
- You need to add the Office365Groups connector to your PowerApps. To do this, go to the Data menu, and select Office 365 Groups. This will add the connector to your PowerApps.
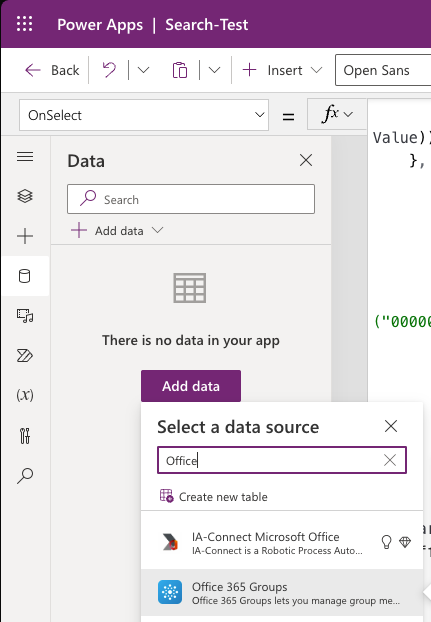
Using Office365Groups.HttpRequest
The Office365Groups.HttpRequest allows you to make HTTP requests to external services from within your PowerApps. This can be useful when you want to retrieve data from an external source, such as the Graph API.
Here’s an example of how you might use Office365Groups.HttpRequest to search for a message by its subject:
|
|
The code above sets a variable named varRequestBody
. The JSON object contains a single property named requests .The object in the array represent the search request and contains two properties: entityTypes and query. The entityTypes property a string that specifies the type of entity to search for. In this case, the entity type specified is message (Email’s). The query property is an object that contains a single property named queryString. The queryString property is a string that specifies the search query to execute. In this case, the search query is subject:Hello
.
|
|
This nice trick I learned from Hiro’s Blog - Encode plain text to Base64 - https://mofumofupower.hatenablog.com/entry/encode_decode
The varRequestBody
variable is then used to generate a Base64-encoded string that represents the request body for an HTTP request. This is done by first converting the varRequestBody variable to binary format, then to Base64 format. The resulting Base64 string is then used as the request body for the HTTP request.
|
|
The HTTP request is sent to the Microsoft Graph API’s search endpoint (https://graph.microsoft.com/v1.0/search/query
) using the Office365Groups.HttpRequest
function. The function takes three arguments: the URL of the endpoint, the HTTP method to use (in this case, POST
), and the request body to send with the request.
This whole code can for example be put in a buttons OnSelect property to send the request when the button is clicked.
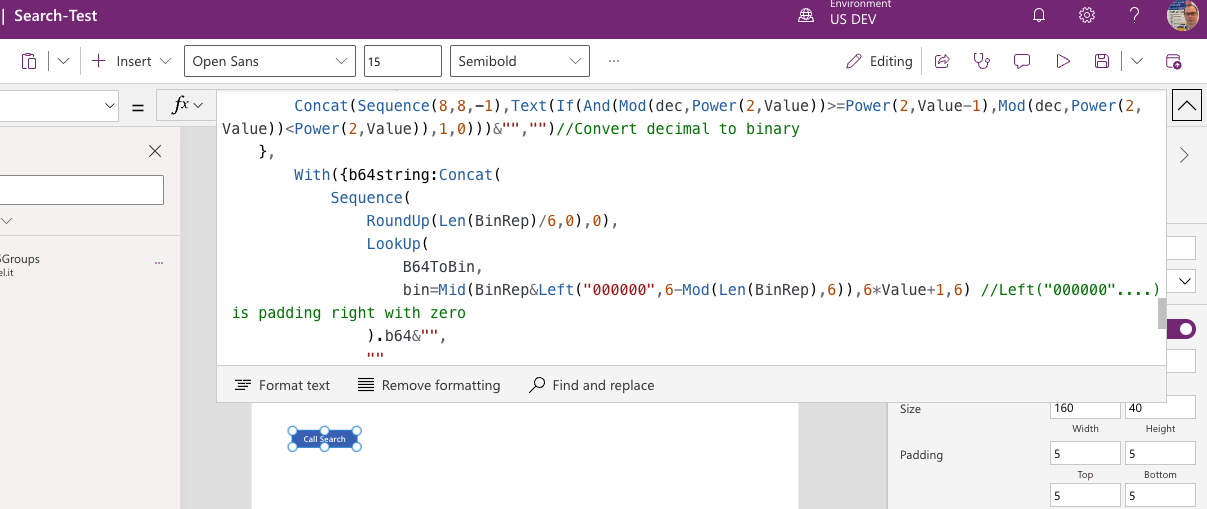
Showing the Results in a Gallery
Once we’ve made our HTTP request and retrieved the result, we can use a gallery to display the results. To do this, we’ll need to insert a gallery control to our App and set the Items
property of the gallery to the following. To be able to work with those results, we need to get the resonse body from the HTTP request. This is done by doing one of the following:
- Make the same call in the Graph Explorer and exam the response body from there
- In PowerApps start the App - Monitor to get the response body from there
|
|
The varResult
variable is containing the response from the API. The response is expected to be a JSON object that contains a property named value
. The value
property is an array of objects that represent the search results.
The code uses the Table
function to convert the varResult.value
array to a table. The resulting table has a single column named Column1
that contains the search results. The Index
function is then used to extract the first element of the Column1
array, which is another table. This table represents the hitsContainers
property of the search results.
The Index
function is used again to extract the first element of the hitsContainers
table, which is another table. This table represents the hits
property of the search results. Finally, the Table
function is used again to convert the hits
table to a table that can be displayed in the output pane of Visual Studio Code.
Next, we’ll need to add a label control to the gallery and set its Text
property to the following:
|
|
In a gallery, each item is represented by a record that contains one or more fields. The ThisItem
function is used to reference the current item in the gallery.
If we look at the output of the Table
function, we can see that each item in the gallery is represented by a record that contains a resource
field. The resource
field is an object that contains a subject
field.
Therefore, ThisItem.Value.resource.subject
is used to extract the subject of the resource from the current search result and display it in the label control.
Conclusion
In this blog post, I’ve shown you how to use Graph API calls to Microsoft Search in PowerApps by using the Office365Groups.HttpRequest and the preview feature “ParseJSON function and untyped objects”. By following these steps, you’ll be able to retrieve data from external sources and use it within your PowerApps. We hope you found this post helpful! Let me know if you have any questions or comments.
Additional Resources
-
Some links to more information about Microsoft Search and the Graph API:
- Microsoft Graph Explorer - https://developer.microsoft.com/en-us/graph/graph-explorer
- Microsoft Graph API documentation - https://learn.microsoft.com/en-us/graph/search-concept-overview
-
For testing / creating search queries:
- SharePoint Search Query Tool - https://github.com/pnp/PnP-Tools (Windows only)
- SP-Editor - Search Microsoft Edge Add-ons for it in the future as the Search part isn’t yet implemented in the meantime get the old Add-on from the Chrome Web Store (Windows, Mac, Linux)